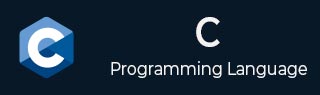
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
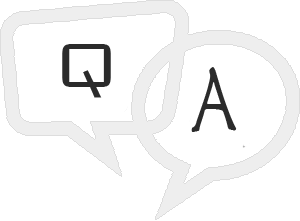
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { int a = 5, b = 3, c = 4; printf("a = %d, b = %d\n", a, b, c); }
Answer : A
Explanation
a=5,b=3 , as there are only two format specifiers for printing.
Q 2 - What is the value of ‘y’ for the following code snippet?
#include<stdio.h> main() { int x = 1; float y = x>>2; printf( "%f", y ); }
Answer : C
Explanation
0, data bits are lost for the above shift operation hence the value is 0.
Q 3 - Linker generates ___ file.
Answer : B
Explanation
(b). Linker links the object code of your program and library code to produce executable.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { fprintf(stdout,"Hello, World!"); }
Answer : A
Explanation
stdout is the identifier declared in the header file stdio.h which is connected to standard output device (monitor).
Q 5 - What is the output of the following program?
#include<stdio.h> main() { char *s = "Abc"; while(*s) printf("%c", *s++); }
Answer : A
Explanation
Loop continues until *s not equal to ‘\0’, hence printing “Abc” where character is fetched first and address is incremented later.
Answer : A
Explanation
#include <stdio.h> int main() { long int decimalNumber,remainder,quotient; int binaryNumber[100], i = 1, j; printf("Enter any decimal number: "); scanf("%ld",&decimalNumber); quotient = decimalNumber; while(quotient!=0){ binaryNumber[i++]= quotient % 2; quotient = quotient / 2; } printf("Equivalent binary value of decimal number %d: ",decimalNumber); for(j = i -1 ;j> 0;j--) printf("%d",binaryNumber[j]); return 0; }
Q 7 - Which library functions help users to dynamically allocate memory?
Answer : C
Explanation
malloc():Allocates an area of memory that is large enough to hold "n” number of bytes and initializes contents of memory to zeroes.
calloc(): Allocates "size" of bytes of memory and contains garbage values.
Q 8 - What do you mean by “int (*ptr)[10]”
A - ptr is an array of pointers to 10 integers
B - ptr is a pointer to an array of 10 integers
Answer : B
Explanation
Explanation: with or without the brackets surrounding the *p, still the declaration says it’s an array of pointer to integers.
Q 9 - What will be the output of the following program?
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Answer : D
Explanation
The above program will return error
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Q 10 - extern int fun(); - The declaration indicates the presence of a global function defined outside the current module or in another file.
Answer : A
Explanation
Extern is used to resolve the scope of global identifier.
To Continue Learning Please Login